Enhancing DataTables in LWC – Part 3 Multi-line Cells
This example shows how we can get a datatable to show a cell with two lines of text. This can help when there are less rows to show but more columns, so you can combine two fields in one cell:
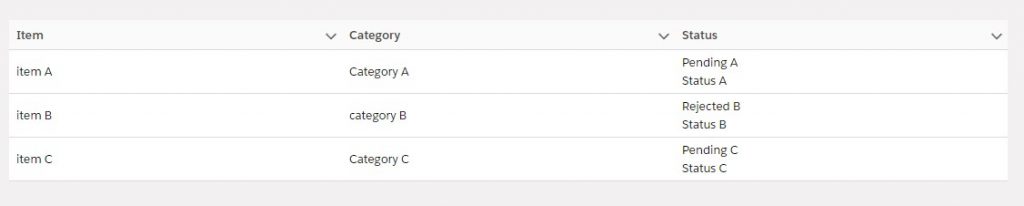
Lets set up a simple demo LWC to show the table – called multiLineTableDemo:
<template>
<c-custom-multi-line-data-table
data={data}
columns={columns}
key-field="item"
hide-checkbox-column
>
</c-custom-multi-line-data-table>
</template>
import { LightningElement } from "lwc";
const sampleData = [
{
id: "1",
itemName: "item A",
category: "Category A",
status: "Pending A",
status1: "Status A"
},
{
id: "2",
itemName: "item B",
category: "category B",
status: "Rejected B",
status1: "Status B"
},
{
id: "3",
itemName: "item C",
category: "Category C",
status: "Pending C",
status1: "Status C"
}
];
const columns = [
{
label: "Item",
fieldName: "itemName"
},
{
label: "Category",
fieldName: "category"
},
{
label: "Status",
type: "customMulti",
typeAttributes: {
line1: { fieldName: "status" },
line2: { fieldName: "status1" }
}
}
];
export default class MultiLineTableDemo extends LightningElement {
data = sampleData;
columns = columns;
}
The above code sets up some columns and test data and then we have a LWC component that would be CustomMultiLineDataTable. The status column has a type of customMulti has the typeAttributes setting a line1 and line2 based on the status and stauts1 fields.
Lets work down a level of detail into the CustomMultiLineDataTable:
<template></template>
import LightningDatatable from "lightning/datatable";
import customMultiLineRow from "./customMultiLineRow.html";
export default class CustomMultiLineDataTable extends LightningDatatable {
static customTypes = {
customMulti: {
template: customMultiLineRow,
standardCellLayout: true,
typeAttributes: ["line1", "line2"]
}
};
}
There is not much to this, we have a blank template and then in the javascript file we are extending the LightningDatatable and defining our customType calls customMulti with a template, standardCellLayout and then typeAttributes.
The second line of the file imports the customMultiLineRow html file which is :
<template>
<c-custom-multi-row
line1={typeAttributes.line1}
line2={typeAttributes.line2}
></c-custom-multi-row>
</template>
This file is in the same directory as the LWC:
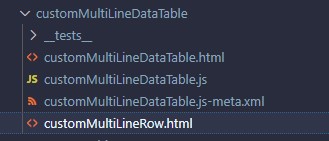
This template refers to the individual cell in another LWC called CustomMultiRow, whihc is the final rendering of the cell (which is super simple):
<template>
<div>{line1}</div>
<div>{line2}</div>
</template>
import { LightningElement, api } from "lwc";
export default class CustomMultiRow extends LightningElement {
@api line1;
@api line2;
}
So we are simply setting up two div elements with line1 and line2.
The final files and arrangement is:
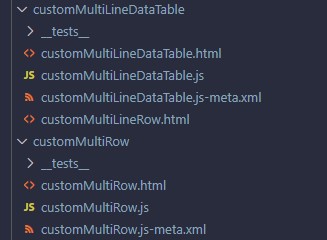
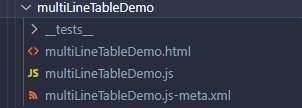