Simple Project – Post 1 – Querying Static Resources
This is the first of short set of posts that will build in to a working example. The overall example will allow the user to select a document from a static resource and then send the static resource as an attachment on an email. This will demonstrate the following:
- How to list the documents in a static resource
- How to present a datatable to the user with a select to move the next screen of the flow
- How to attach a specific static resource to an email and send
The first stage is to create a static resource to upload. This link is a zipped file of 5 pdf documents called Document 1.pdf, Document 2.pdf, Document 3.pdf, Document 4.pdf and Document 5.pdf. These have been zipped up with WinRAR but using the compression method as Store. We will be using a library to unzip the files and see what is in them so setting the compression method as store seems to help the processing CPU required.
This is uploaded as a static resource called ‘Attached_Documents’ :
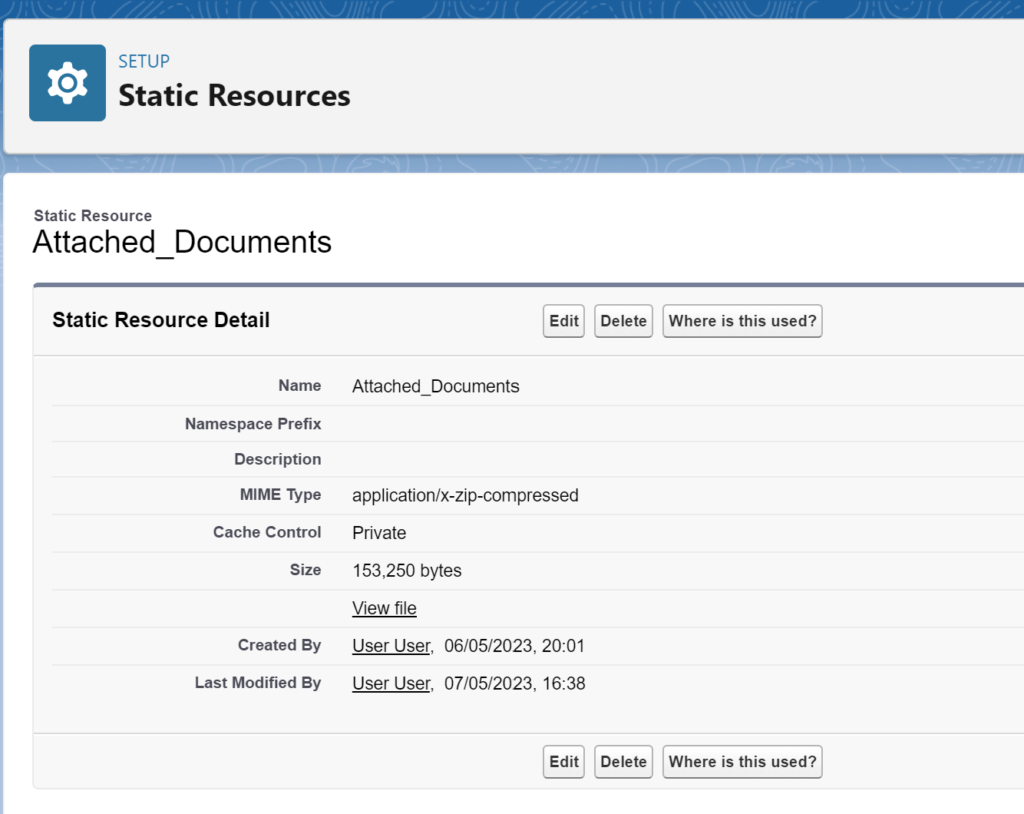
From APEX we can query static resources via the StaticResource object. The code below will locate the static resource and return a Blob of the body. The Blob is a binary object and is the zipped up file. We will need to query this zipped file to get a list of the documents in it:
List<StaticResource> staticResource = [Select id, name, body from StaticResource
where name = 'Attached_Documents'];
if (staticResource == null)
return 'Error';
Blob staticBody = staticResource[0].body;
We can use an open source library to query the zipped file. This is called Zippex and can be viewed at https://github.com/pdalcol/Zippex. Care should be taken with using external libraries – there are additional security risks in using external libraries. The instructions to install are simple in that 4 classes need to be uploaded to your environment.
To use we pass in the zipped blob to the library and there are a range of commands that we can use. Firstly we will query to get the list of files in the zipped file. So the code below will take
Zippex sampleZip = new Zippex(staticBody);
Set <String> fileNames = sampleZip.getFileNames();
We have two choices at this point, we eventually want to present this back to our user in a datatable so we can either set up the JSON file as we need in apex or pass back as is to the lwc and do the processing there. We will do this in apex and so we will need a JSON file that will look like this:
[
{"document" : "Document 1.pdf"},
{"document" : "Document 2.pdf"},
{"document" : "Document 3.pdf"},
{"document" : "Document 4.pdf"},
{"document" : "Document 5.pdf"}
]
This will allow a datatable with the fieldname document to display the 5 rows listing the names of the files. To do this will create a JSON object and then pass through the set of files and create the object we need. :
JSONGenerator jsonReturn =JSON.createGenerator(true);
jsonReturn.writeStartArray();
for (String fileName : fileNames)
{
jsonReturn.writeStartObject();
jsonReturn.writeStringField('document', fileName);
jsonReturn.writeEndObject();
}
jsonReturn.writeEndArray();
So our Apex file is :
public with sharing class staticController {
@AuraEnabled(cacheable=true)
public static string getStaticDescription() {
List<StaticResource> staticResource = [Select id, name, body from StaticResource
where name = 'Attached_Documents'];
if (staticResource == null)
return 'Error';
Blob staticBody = staticResource[0].body;
Zippex sampleZip = new Zippex(staticBody);
Set <String> fileNames = sampleZip.getFileNames();
JSONGenerator jsonReturn =JSON.createGenerator(true);
jsonReturn.writeStartArray();
for (String fileName : fileNames)
{
jsonReturn.writeStartObject();
jsonReturn.writeStringField('document', fileName);
jsonReturn.writeEndObject();
}
jsonReturn.writeEndArray();
return jsonReturn.getAsString();
}
}
In the next post we will present these options to a user in a flow.