Adventures in Salesforce and DataWeave (Beta) Part 2
In Part 1 we set up a scratch org and had a look at how DataWeave works. Lets now get this working in our apex.
We need a folder called dw that the DataWeave file will be added to
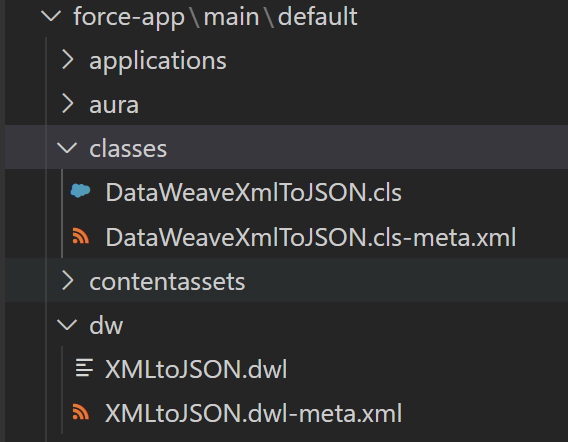
The XMLtoJSON.dwl has our DataWeave file
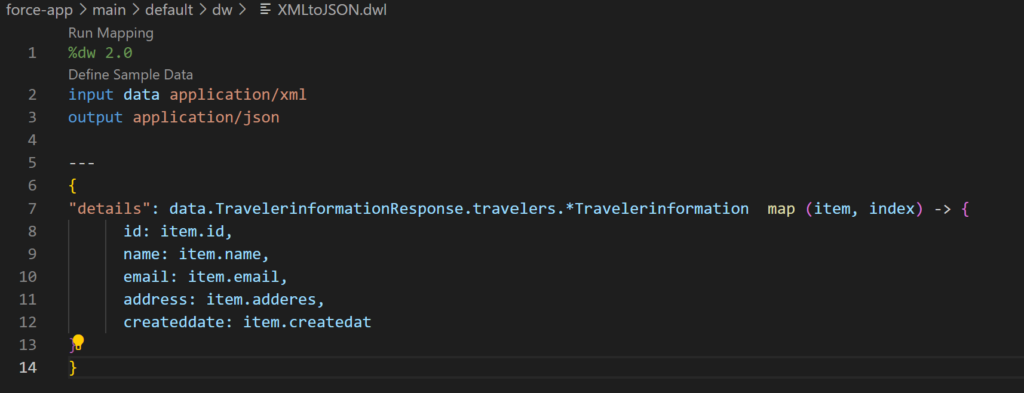
and the XMLtoJSON.dwl-meta.xml is the description of the XMLtoJSON.dwl

With this in place we can write our Apex class to firstly load the DataWeave file called XMLtoJSON and then pass in the XML string referenced by the mapping ‘data’ string
public static string ProcessXMLtoJSON() {
string XML = CallWebSite();
Dataweave.Script dwscript = DataWeave.Script.createScript(
'XMLtoJSON'
);
DataWeave.Result result = dwscript.execute(
new Map<String, Object>{ 'data' => xml }
);
string jsonResult = result.getValueAsString();
return jsonResult;
}
This is all we need to do read in an XML file, transform with new field names, translate to JSON. This would be a pretty complex and difficult thing to do without DataWeave.
Lets bring this data back to a LWC component. Firstly lets enable to class to be accessed by a LWC wire adapter:
@AuraEnabled(cacheable=true)
Now we can create a LWC component called processXMLtoJSON with the following:
<template>
<lightning-datatable
data={data}
columns={columns}
key-field ="id"
>
</lightning-datatable>
</template>
import { LightningElement, wire } from 'lwc';
import processXMLtoJSON from "@salesforce/apex/DataWeaveXmlToJSON.ProcessXMLtoJSON";
const columns = [
{
label: "Id", fieldName: "id",
},
{
label: "Name", fieldName: "name"
},
{
label: "Email", fieldName: "email"
},
{
label: "Address", fieldName: "address"
},
{
label: "Created Date", fieldName: "createddate"
},
];
export default class DataWeaveTable extends LightningElement {
data;
columns = columns;
@wire(processXMLtoJSON)
getprocessXMLtoJSON(result) {
if (result.data) {
const jsonObject = JSON.parse(result.data);
this.data = jsonObject.details;
}
if (result.error) {
console.log(result.error);
}
}
}
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>56.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__HomePage</target>
</targets>
</LightningComponentBundle>
Adding this to a home page we should get the following:
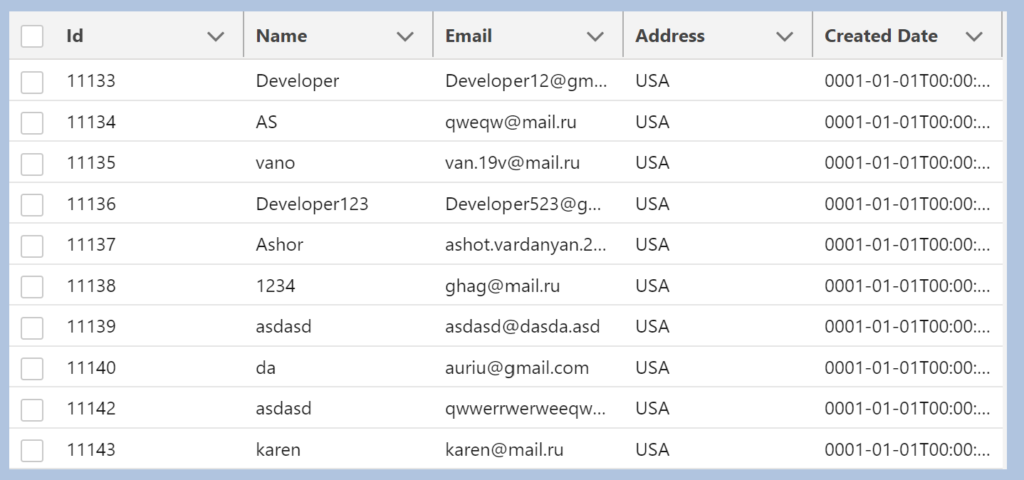
However doing this will expose the biggest weakness of DataWeave – it is not particularly fast. Hopefully at the moment as we are in Beta that when we get to GA then performance might improve.
Part 3 will move on to look at CSV files